GAMES202第二课
基本渲染管线
- 体在空间中的表示 – 点,点和点之间的连接关系
- 顶点处理 MVP
- 图元装配和光栅化
- 片元处理
- 输出合并
型(Blinn-Phong等等)在光线的弹射和阴影的表现上并不是很好(并不是100%真实,在全局和间接光照上处理的不好)
好处是GPU上跑很快
纹理映射和插值–重心坐标
OpenGL(GLSL)
OpenGL介绍
OpenGL是一个API的集合,是CPU运行的负责调用GPU工作任务的。
优势:
坏处:
在每个pass中进行的过程
- 放置物体和模型
- 模型声明
- 模型摆放 – model transformation
- VBO:GPU中的一块区域用于存储模型与.obj文件特别相似
- 设置相机位置
- 视口变换 – view transformation
- 在OpenGL中创建framebuffer
- 设置画布
- 这里OpenGL可以multiple render target
- 垂直同步,双重缓冲,三重缓冲,目的都是为了防止画面撕裂,撕裂原因是在帧缓冲中上一帧画面渲染到一般又渲染了下一帧
- 画 – shading
- vertex
- 对顶点进行插值
- 图元装配光栅化
- fragment
- 片段着色
- lighting
- 接着画,画多次 – multiple passes
- shadowmap的作法
- 计算光源能看到什么
- 再通过camera来计算这些物体能不能被light看到,不能就是在阴影中
Shader setup步骤
- 创建shader
- 编译shader
- 将shader加载到程序中(attach program)
- 连接程序(link program)
- 使用程序(use program)
Vertex Shader(顶点着色器)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
|
attribute vec3 aVertexPosition;
attribute vec3 aNormalPosition;
attribute vec3 aTextureCoord;
uniform mat4 uModeViewMatrix; uniform mat4 uProjectionMatrix;
varying highp vec2 vTextureCoord; varying highp vec3 vFragPos; varying highp vec3 vNormal;
void main(void){ vFragPos = aVertexPosition; vNormal = aNormalPosition; gl_Position = uProjectionMatrix * uModelViewMatrix * vec4(aVertexPosition, 1.0); vTextureCoord = aTextureCoord; }
|
Fragment shader
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| uniform sampler2D uSampler;
uniform vec3 uKd; uniform vec3 uKs; uniform vec3 uLightPos; uniform vec3 uCameraPos; uniform float uLightIntensity; uniform int uTextureSample;
varying highp vec2 vTextureCoord; varying highp vec3 vFragPos; varying highp vec3 vNormal;
void main(void){ vec3 color; if(uTextureSample == 1){ color = pow(texture2D(uSampler, vTextureCoord).rgb, vec3(2.2)); }else{ color = uKd; } vec3 ambient = 0.05 * color; vec3 lightDir = normalize(uLightPos - vFragPos); vec3 normal = normalize(vNormal); float diff = max(dot(lightDir, normal), 0.0); float light_atten_coff = uLightIntensity / length(uLightPos - vFragPos); vec3 diffuse = diff * light_atten_coff * color; vec3 viewDir = normalize(uCameraPos - vFragPos); float spec = 0.0; vec3 reflectDir = reflect(-lightDir, normal); spec = pow(max(dot(viewDir, reflectDir), 0.0), 35.0); vec3 specular = uKs * light_atten_coff * spec; gl_FragColor = vec4(pow((ambient + diffuse + specular), vec3(1.0/2.2)), 1.0); }
|
渲染方程
在渲染中是最为重要的
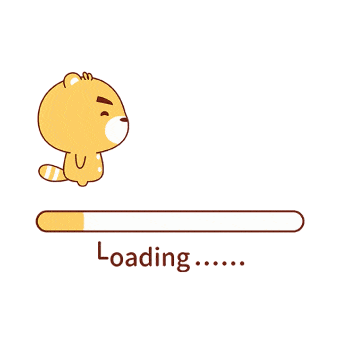
意思解释:
- outgoing radiance:当前点发出的radiance
- emission:点的自发光
- BRDF * cosθ * dw:BRDF乘上cosθ然后乘以其他地方打到这个点上的radiance
- cosθ * dw: 是将radiance转换成irradiance
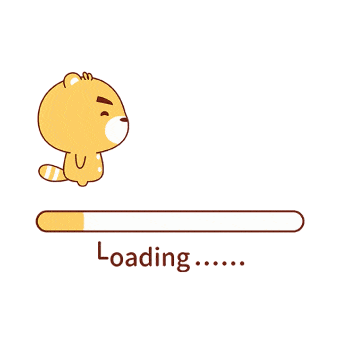
V(p, w):表示点是否可以接收到当前传过来的那束光